PHP 7 Scalar Type Declarations-Section-1
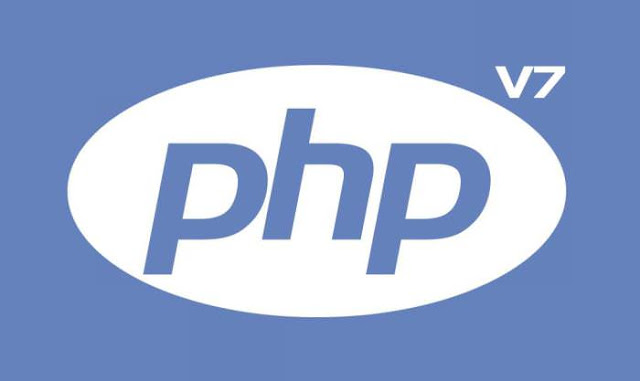
Introduction
In the previous tutorial, we looked at what PHP 7 is and what it can do for us. This tutorial will look at scalar type declarations and a simple practical example. Scalar type declaration is sometimes called "type hinting". It basically allows us to specify the data type of a variable. For example, if you want to sum two integer values, you can explicitly tell PHP to treat the parameters as integers instead of letting PHP decide that for you.Topics to be covered
We will cover the following topics- Tutorial Pre-requisites
- Advantages of Scalar Type Declarations
- Declarations Techniques
- None
- Coercive
- Strict
- PHP 7 Scalar Type Declaration Example
Tutorial Pre-requisites
For you to successfully complete this tutorial, you should have/know the following.- This tutorial assumes that you know the basics of PHP.
- You have an IDE such as NetBeans or a text editor i.e. Sublime Text, Brackets etc.
- You have PHP 7 and a web server already installed and running.
- You have a modern web browser
- You read the previous tutorial that introduces PHP 7. This is optional but highly recommended.
Advantages of Scalar Type Declarations
Specifying the data type has a number of advantages. Let’s look at some of these advantages- Communicates the intent – PHP may be able to figure out the data type and convert it when executing the code but what about other programmers reading your code? It makes it easier for them to follow the code if you specify the data type of the function. In the absence of such explicit declaration, one will have to follow the function to see what type of argument the function may be expecting.
- Precise results –
Let me explain this with an example. Suppose you have a function that
accepts two arguments and sums them up. If you pass the arguments
$a = 6
, and$b = "x"
. The sum is6
. If you multiple$a
and$b
, then you will get0
as the result. This is because the sting"x"
is evaluated to0
and the computation goes smoothly. In a financial system, this can lead to loss of income. Type hinting throws an error if the conversion fails as in the above example. - More precise errors –
The immediate above example (assuming scalar hinting is used) will give
us the following fatal error message in PHP 7 Fatal error:
"Uncaught TypeError: Argument 2 passed to sum () must be of the type integer, string given,..."
. PHP 7 allows you to catch Errors in a try block to avoid fatal errors. We will cover error handling in more detail in another tutorial. - Write clean less code – The beauty of programming is if something does not exist out of the box, you can always write your own workaround. This leads to more unnecessary code. Type hinting removes the burden of writing functions that check the data type of arguments before processing.
- Other advantages – if you know an advantage that is not listed in the above list, use the comments section to share with others.
Declarations Techniques
Type hinting in PHP 7 is done in three ways namely;- None – This is the good ol way of doing it. You assign a value to a variable and PHP determines the data type based on the operation that is done on the data type.
- Coercive – This methodology allows you to specify the data type. Let’s say you have a function that accepts an integer
int $a
argument. Passing in a value of"8"
is acceptable. Coercive technical will convert the string value8
to anint
then perform the desired operation on the data. If you pass in a value such as"x"
, this will throw an error because the value"x"
cannot be coerced into an integer. - Strict
– As the name suggests, the argument value passed in must exactly be
the same as the specified type. This is the equivalent of strong typing.
Let me explain this with the aid of an example. Suppose you have a
function that accepts an arguments
int $x
. Passing in a value of"8"
for the argument$x
throws an error. This is because the passed in value "8" is of string data. PHP will not try to convert the value for you like we did using coercion.
PHP 7 Scalar Type Declaration Example
Now that we have laid a good foundation, let’s build a hut on it. In this section, we will create a simple project that demonstrates the implement of scalar type declaration in PHP 7.Let’s assume we are developing a simple program that multiplies two numbers and returns the result.
Step 1 – Create a new project
I am using XAMPP on windows so I will just create a new directoryphp7-scalar-types
in C:\htdocs\xampp
Step 2 – Create the following three files
- none.php
- coercive.php
- strict.php
Step 3 – Code for implicit (None) declaration
Opennone.php
and add the following code<?php
function getSubTotal($quantity, $price){
return $quantity * $price;
}
echo "String parameter values returned : " . getSubTotal("3","2100");
echo "<br>";
echo "String parameter values (quantity as letter) returned : " . getSubTotal("x","2100");
echo "<br>";
echo "Integer parameter values returned : " . getSubTotal(3,2100);
- The above function
getSubTotal($quantity, $price){…}
accepts two parameters$quantity
and$price
to calculate the sub total echo…. getSubTotal(x,y)
tests different data types as input to the function and displays the result in the web browser.
http://localhost/php7-scalar-types/none.php
- String parameter values returned : 6300
- String parameter values (quantity as letter) returned : 0
- Integer parameter values returned : 6300
- The first function call used arguments of string data types but we got the correct answer
- The second function call used arguments of string data types and deliberately passed in a letter. The letter was evaluated to 0 and we got the wrong answer.
- The third function call used the proper data types and we got the correct answer.
Step 4 – Code for coercive.php
- Open
coercive.php
- Add the following code
<?php function getSubTotal(int $quantity, int $price){ return $quantity * $price; } echo "String parameter values returned : " . getSubTotal("3","2100"); echo "<br>"; echo "String parameter values (quantity as letter) returned : " . getSubTotal("x","2100"); echo "<br>"; echo "Integer parameter values returned : " . getSubTotal(3,2100);
function getSubTotal(int $quantity, int $price){…}
the functiongetSubTotal(x,y)
accepts two arguments ofint
data types. This means any argument value passed in will be converted to aninteger
before performing any operations on the data.int $quantity, int $price
theint
at the beginning declares the value data type
http://localhost/php7-scalar-types/coercive.php
- String parameter values returned : 6300
Fatal error: Uncaught TypeError: Argument 1 passed to getSubTotal() must be of the type integer, string given
, called in C:\xampp\htdocs\php7-scalar-types\coercive.php on line 9 and defined in C:\xampp\htdocs\php7-scalar-types\coercive.php:3 Stack trace: #0 C:\xampp\htdocs\php7-scalar-types\coercive.php(9): getSubTotal('x', '2100') #1 {main} thrown in C:\xampp\htdocs\php7-scalar-types\coercive.php on line 3
- We passed in string values but PHP coerced them into integers and gave us the correct answer
- A fatal error occurred because the string value
'x'
could not be coerced into an integer.
Step 5 – Code for strict.php
- Open strict.php
- Add the following code
<?php declare(strict_types=1); function getSubTotal(int $quantity, int $price){ return $quantity * $price; } echo "Integer parameter values returned : " . getSubTotal(3,2100); echo "<br>"; echo "String parameter values returned : " . getSubTotal("3","2100"); echo "<br>"; echo "String parameter values (quantity as letter) returned : " . getSubTotal("x","2100");
declare(strict_types=1);
sets strict typing to true. The default value is 0. This declaration should be the first line in a file or else PHP will throw an error. Strict types is applied on a file basis. It is not made global.
http://localhost/php7-scalar-types/strict.php
- Integer parameter values returned : 6300
Fatal error: Uncaught TypeError: Argument 1 passed to getSubTotal() must be of the type integer, string given
, called in C:\xampp\htdocs\php7-scalar-types\strict.php on line 11 and defined in C:\xampp\htdocs\php7-scalar-types\strict.php:5 Stack trace: #0 C:\xampp\htdocs\php7-scalar-types\strict.php(11): getSubTotal('3', '2100') #1 {main} thrown in C:\xampp\htdocs\php7-scalar-types\strict.php on line 5
- The first function call returned the correct result. We passed in integer data type values
- The second call passes in string values that can be converted into integers. PHP throws a fatal line because the expected value should be an integer and not a string.
Summary
Scalar Type Declaration helps us to write better code when dealing with variables and PHP still offers us the flexibility of weak typing.What’s Next?
The next tutorial will look at PHP 7 Scalar Type Declarations. In a nutshell, you can now have the option specify the variable data type when declaring variables.If you found this tutorial useful, support us by using the social media buttons to like and share the tutorial. If you didn’t find it useful, please use the comments section below to let us know how we can do better next time.