PHP 7 Return Type Declarations-Section-2
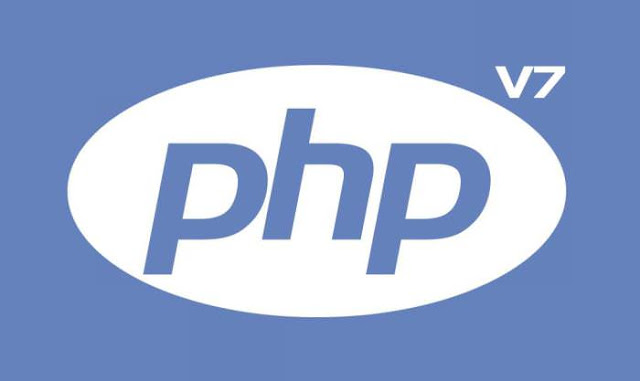
Introduction
In the previous tutorial, we looked at scalar type declarations. We focused on the data types of arguments that we were passing to functions. In this tutorial, we will look at how we can specify the data type of the result that a function returns.Topics to be covered
We will cover the following topics- Tutorial pre-requisites
- Type Declarations - Valid Types
- Return Type Declarations Techniques
- None
- Coercive
- Strict
- PHP 7 Return Type Declaration Example
Tutorial Pre-requisites
For you to successfully complete this tutorial, you should have/know the following.- This tutorial assumes that you know the basics of PHP.
- You have an IDE such as NetBeans or a text editor i.e. Sublime Text, Brackets etc.
- You have PHP 7 and a web server already installed and running.
- You have a modern web browser
- You read the previous tutorial that introduces PHP 7. This is optional but highly recommended.
Type Declarations - Valid Types
The following are the supported types- class / interface
- self
- array
- callable
- bool
- float
- int
- string
Return Type Declarations Techniques
The rules for declaring return types are not different from the ones for declaring scalar types.- None – no type is explicitly specified
- Coercive – coerces the result to the specified type
- Strict – the result must be of the specified data type. No exceptions
PHP 7 Return Type Declaration Example
In this section, we will create a simple project that demonstrates the usage of return types in PHP 7. We will look at two examples that use coercive and strict types.Step 1 – Create a new project
I am using XAMPP on windows so I will just create a new directoryphp7-return-types
in C:\htdocs\xampp
Step 2 – Create the following three files
- coercive.php
- strict.php
Step 3 – Code for coercive.php
Opencoercive.php
Add the following code
http://localhost/php7-return-types/coercive.php
String parameter values returned : 6300 of data type integer
- we passed in string parameters that were coerced into integers. Therefore, the result returned result is of integer data type.
Modify the above
getSubTotal(…)
function to the followingfunction getSubTotal(int $quantity, int $price) : string {
return $quantity * $price;
}
function getSubTotal(int $quantity, int $price) : string {…}
the full colon followed by the desired scalar type is used to define the data type that the function should return. In our case, our function will return a string.
Load the following URL into your web browser
http://localhost/php7-return-types/coercive.php
String parameter values returned : 6300 of data type string
- The data type returned this time is a string. PHP converted the integer result into a string before the returning the result.
Step 4 – Code for strict.php
- Open
strict.php
- Add the following code
<?php declare(strict_types=1); function getSubTotal($quantity, $price) : int { return $quantity * $price; } echo "String parameter values returned : " . getSubTotal(3.1,2100.7);
•
declare(strict_types=1);
activates strict typing. • function getSubTotal($quantity, $price) : int {…}
sets int
as the return type. Note we did not specify the data type for the $quantity
and $price
.We deliberately passed in parameter values of float data type. The result will also be a float and PHP will throw an error because its expecting the result to be an integer and not float
Load the following URL into your web browser
http://localhost/php7-return-types/strict.php
Fatal error: Uncaught TypeError: Return value of getSubTotal() must be of the type integer, float returned in C:\xampp\htdocs\php7-return-types\strict.php:6 Stack trace: #0 C:\xampp\htdocs\php7-return-types\strict.php(9): getSubTotal(3.1, 2100.7) #1 {main} thrown in C:\xampp\htdocs\php7-return-types\strict.php on line 6
- Return value of
getSubTotal()
must be of the type integer, float returned in… tells us the expected return type should an integer and not float
Summary
Return type declarations allow us to specify the expected data type that our function should return. The rules for return type declarations are very similar to the rules for scalar type declarations.What’s Next?
The next tutorial will look at new operators in PHP 7 (Spaceship and Null Coalescing). In a nutshell, you can now have the option specify the variable data type when declaring variables.If you found this tutorial useful, support us by using the social media buttons to like and share the tutorial. If you didn’t find it useful, please use the comments section below to let us know how we can do better next time.