Laravel 5 Faker Tutorial
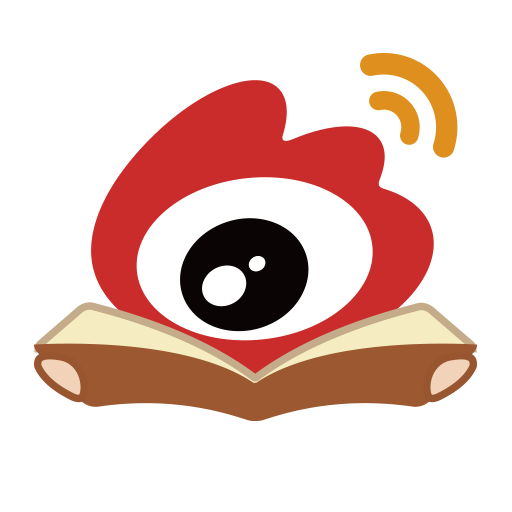
Introduction
Database seeding is one of those cool features in Laravel that we all love. However, adding multiple records to the database writing them one by one kind of sucks and takes away a lot of valuable time. This is where faker library comes in.Faker is a PHP library used to generate fake data for testing purposes. It can be used to generate all sorts of data.
Topics to be covered
We will cover the following topics in this tutorial- How to install Faker in Laravel
- Laravel Faker Basic Usage
- Laravel Database Seeding using faker
How to install Faker in Laravel
Laravel installs faker by default. You don’t have to manually. You all you to do is start using it.Faker can be used to generate the following data types
- Numbers
- Lorem text
- Person i.e. titles, names, gender etc.
- Addresses
- Phone numbers
- Companies
- Text
- DateTime
- Internet i.e. domains, URLs, emails etc.
- User Agents
- Payments i.e. MasterCard
- Colour
- Files
- Images
- uuid
- Barcodes
- Miscellaneous
Faker Basic Usage
Let’s start by creating a new Laravel project.Run the following composer command
composer create-project laravel/Laravel larafaker
/app/Http/routes.php
Add the following route
Route::get('/customers',function(){
$faker = Faker\Factory::create();
$limit = 10;
for ($i = 0; $i < $limit; $i++) {
$faker->name . ', Email Address: ' . $faker->unique()->email . ', Contact No' . $faker->phoneNumber . '<br>';
}
});
$faker = Faker\Factory::create();
creates a variable for Faker Factory$limit = 10;
sets the for loop limitfor ($i = 0; $i < $limit; $i++) {…}
executes the same code a number of times as specified by the limit variableecho $faker->name . ', Email Address: ' . $faker->unique()->email . ', Contact No' . $faker->phoneNumber . '<br>';
uses faker to generate a name, unique email address and contact number and display them in the browser.
http://localhost/larafaker/public/customers
Prof. Luciano Johnson Sr., Email Address: mGibson@hotmail.com, Contact No1-325-254-2558x5828
Issac Turcotte, Email Address: Amy77@Cruickshank.com, Contact No+05(8)9008932202
Alfreda Lesch, Email Address: fWalsh@yahoo.com, Contact No838.460.2999x435
Moses Hilll, Email Address: Mable.Frami@Fritsch.com, Contact No967-214-4263x6676
Mr. Emmett White, Email Address: Kihn.Tess@gmail.com, Contact No+55(7)8197619484
Kristopher Luettgen IV, Email Address: rSkiles@gmail.com, Contact No421-059-9607x2059
Jammie Steuber V, Email Address: Hane.Guiseppe@hotmail.com, Contact No764.271.1083
Narciso Pouros PhD, Email Address: Dare.Jeffery@Reichert.biz, Contact No+06(5)1030483446
Prof. Paul Mills, Email Address: jKertzmann@Mueller.com, Contact No091.167.1263x208
Dr. Lucie Pollich, Email Address: Vivian.White@Nader.com, Contact No03197415831
Laravel Database Seeding using Faker
Let’s start with database configurations. Create a database in MySQL called faker.Open
/.env
file in the root directory of the project.Locate the following lines
DB_HOST=localhost
DB_DATABASE=homestead
DB_USERNAME=homestead
DB_PASSWORD=secret
DB_HOST=localhost
DB_DATABASE=faker
DB_USERNAME=root
DB_PASSWORD=melody
Let’s now create a database table via migrations that we will populate with data from faker.
php artisan make:migration customers
/database/migrations/2015_10_01_130021_customers.php
Note: your migration time stamp will be different from the one shown here. It is based on the current time on the server.Modify the code to the following
<?php
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Database\Migrations\Migration;
class Customers extends Migration
{
/**
* Run the migrations.
*
* @return void
*/
public function up()
{
Schema::create('customers', function (Blueprint $table) {
$table->increments('id');
$table->string('name');
$table->string('email')->unique();
$table->string('contact_number');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*
* @return void
*/
public function down()
{
Schema::drop('customers');
}
}
Run the following artisan command to run the migration
php artisan migrate
Migrated: 2015_10_01_130021_customers
Run the following artisan command to create a seeder
php artisan make:seeder CustomersTableSeeder
Seeder created successfully
/database/seeds/CustomersTableSeeder.php
Modify the code to the following
<?php
use Illuminate\Database\Seeder;
class CustomersTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public function run()
{
$faker = Faker\Factory::create();
$limit = 33;
for ($i = 0; $i < $limit; $i++) {
DB::table('customers')->insert([ //,
'name' => $faker->name,
'email' => $faker->unique()->email,
'contact_number' => $faker->phoneNumber,
]);
}
}
}
- The above code inserts 33 records into customers’ table using data generated by faker.
php artisan db:seed --class=CustomersTableSeeder
Run a SELECT query in MySQL for customers table, you will get results similar to the following.
