Laravel 5 REST API
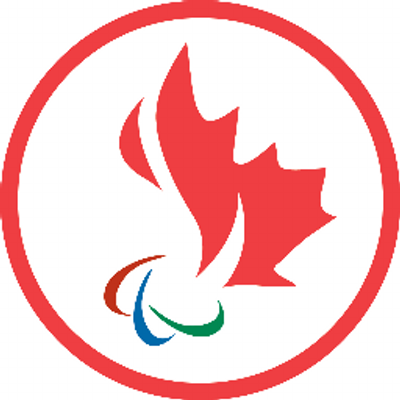
Introduction
Almost all successful internet based companies have APIs. API is an acronym for Application Programming Interface. APIs allows different systems to communicate with one another. Let’s say you have developed an android application for our online store. The API can be used to retrieve data from the online store and display it in the mobile application. The API can also be used to process orders from remote clients such as mobile applications, other websites etc.Topics to be covered
We will cover the following topics- What is a RESTful API?
- REST API Best Practices
- Larashop API
What is a RESTful API?
REST is the acronym for Representational State Transition. It is a software architectural design for building scalable web services. REST APIs allow systems to communicate over HTTP using HTTP verbs. HTTP GET is used to get resources, POST used to create new resources, PUT to update existing ones and DELETE to delete existing resources.REST API Best Practices
This is a summary from the blog post we posted on Kode Blog 10 REST API Design Best Practices That Will Make Developers Love Your API. Read the article for detailed explanations of this summary.- Best Practice # 1: Use HTTP VERBS to determine action to be taken
- Best Practice # 2: API Versioning
- Best Practice # 3: Use plurals to describe resources
- Best Practice # 4: Use query strings to build relations
- Best Practice # 5: Partial responses
- Best Practice # 6: Response Codes and Error Handling
- Best Practice # 7: Limit the number of request in a given time period from the same IP Address
- Best Practice # 8: Use OAuth latest version for authentication
- Best Practice # 9: use JSON as the default
- Best Practice # 10: Cache GET results for less frequently changing data
Larashop API
For now, we will only display the products and categories. Our API will implement basic authentication only. Future tutorial updates will include more functionality.Our API will have the following URLs. All the URLs will use the HTTP verb GET
S/N | Resource | URL | Description | Status Code |
---|---|---|---|---|
1 | Product | /api/v1/products | List products | 200 |
2 | Product | /api/v1/products/1 | List product with id 1 | 200 |
3 | Category | /api/v1/categories | List categories | 200 |
4 | Category | /api/v1/categories/1 | List category with id 1 | 200 |
- Open
/app/Http/routes.php
- Add the following routes
// API routes...
Route::get('/api/v1/products/{id?}', ['middleware' => 'auth.basic', function($id = null) {
if ($id == null) {
$products = App\Product::all(array('id', 'name', 'price'));
} else {
$products = App\Product::find($id, array('id', 'name', 'price'));
}
return Response::json(array(
'error' => false,
'products' => $products,
'status_code' => 200
));
}]);
Route::get('/api/v1/categories/{id?}', ['middleware' => 'auth.basic', function($id = null) {
if ($id == null) {
$categories = App\Category::all(array('id', 'name'));
} else {
$categories = App\Category::find($id, array('id', 'name'));
}
return Response::json(array(
'error' => false,
'user' => $categories,
'status_code' => 200
));
}]);
Route::get('/api/v1/products/{id?}', ['middleware' => 'auth.basic', function($id = null)
defines a RESTful URL for version 1 of the API. The requested resource is Products. {id?} specifies an optional parameter. The id is used to retrieve a single product. The API uses basic authentication- The routes are calling the respective models to retrieve the data from the database.
return Response::json(…)
returns the results in JSON format.
http://localhost/larashop/public/api/v1/products
User a registered email address and password from the previous tutorial on Authetication You will get the following results